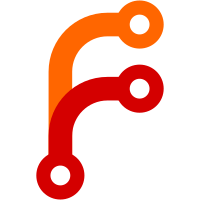
* Refactor numeric decode regex to module attribute, avoid recreating it with every call. * Use character table also when encoding. This results in some compile warns, but the code works as expected. Removing the warnings is for TODO. * Bump elixir version to 0.11.x * Write tests for encoding and decoding
72 lines
2.5 KiB
Elixir
72 lines
2.5 KiB
Elixir
Code.require_file "test_helper.exs", __DIR__
|
||
|
||
defmodule ExcoderTest do
|
||
use ExUnit.Case
|
||
|
||
test "Decode single entity" do
|
||
assert(ExCoder.decode("&") == "&")
|
||
end
|
||
|
||
test "Decode numeric entity" do
|
||
assert(ExCoder.decode("ᄀ") == "ᄀ")
|
||
end
|
||
|
||
test "Decode hexadecimal entity" do
|
||
assert(ExCoder.decode("ģ") == "ģ")
|
||
end
|
||
|
||
test "Decode invalid hexadecimal" do
|
||
assert(ExCoder.decode("h23;") == "h23;")
|
||
end
|
||
|
||
test "Decode invalid numeric" do
|
||
assert(ExCoder.decode("Džg3;") == "Džg3;")
|
||
end
|
||
|
||
test "Decode HTML" do
|
||
assert(ExCoder.decode("""
|
||
<div class='line' id='LC9'> <span class="ss">address:</span> <span class="s2">"chat.eu.freenode.net"</span><span class="p">,</span></div><div class='line' id='LC10'> <span class="ss">
|
||
"""
|
||
) == """
|
||
<div class='line' id='LC9'> <span class="ss">address:</span> <span class="s2">"chat.eu.freenode.net"</span><span class="p">,</span></div><div class='line' id='LC10'> <span class="ss">
|
||
"""
|
||
)
|
||
end
|
||
|
||
test "Decode invalid case" do
|
||
assert(ExCoder.decode("&AOPF;") == "&AOPF;")
|
||
end
|
||
|
||
|
||
|
||
|
||
|
||
test "Encode simple text" do
|
||
assert(ExCoder.encode("foo and bar") == "foo and bar")
|
||
end
|
||
|
||
test "Encode linebreak" do
|
||
assert(ExCoder.encode("
|
||
foo
|
||
and
|
||
bar
|
||
") == "
|
||
foo
|
||
and
|
||
bar
|
||
")
|
||
end
|
||
|
||
test "Encode scandinavian characters" do
|
||
assert(ExCoder.encode("Hääyöaie liittyy öylättiin, sanoi Åke.") == "Hääyöaie liittyy öylättiin, sanoi Åke.")
|
||
end
|
||
|
||
test "Encode apple signs" do
|
||
assert(ExCoder.encode("⌘⌥⇧ and the last one is ⎋") == "⌘⌥⇧ and the last one is ⎋")
|
||
end
|
||
|
||
test "Encode decode roundtrip with very difficult unicode (HE COMES)" do
|
||
zalgo = "Z͉̘͕͐ḁ̗̕ͅl̟̥̳̞̞͔ͬ̔̂̋̾gͮͫ̓̓̕o͎͉̹͕͌͂̍͐̌̋ ͕̖͖ͯĩ͇̹̬̤͕̟ͭ̇ș̼̠̹̒̂ͭͯ̓ ͉̪͍͚̗̟͚ḟ̴̙̟̯͚̭̳̼̈́û̔ͥ̒nͥͩͭ̎̃!̫͔ ̲̯̰̰̗͔ͪ͝R̭͉̬͓̜͉ͮͬͤ̃ͯ͊a̡̩̍͊i̖̺̝̻ͦ̋̾͑͌̇ņ͍̖̟͚͓ͧ͒b̻̹͉͉̘̎̄ͬ̆͗͠ơ̮̱͉͓̗̝̯ẅ͍̱́̋ͧͭ̾̐̇s̯͓̦͓̦͇͚̎ͦ̌͐̾ ̟̞̑̉̈̎̈́l̯͉̯ͭ͡ȏ̟͙̯̞̫̳̮͒ͭ̎͆l̼͒ͩ̌̀͒l̒͒ͭ̌ͩ̎͘ͅi̝̲ͬ̋̾̉̋p̢̣͉͒̾͗ͨͯö͙̳̘̜̓ͣ͒́̿ͫͅp̵͔͎͓sͅ.̶̹͙̼̺ͧ͂̒"
|
||
assert(ExCoder.decode(ExCoder.encode(zalgo)) == zalgo)
|
||
end
|
||
end
|